Three Level Image Password Authentication System Php
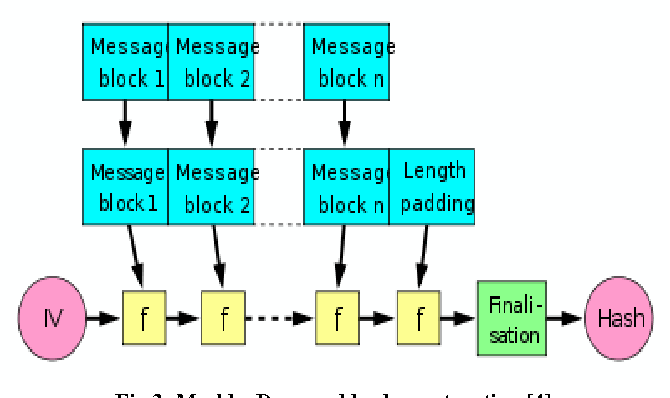
Creating a three-level image password authentication system using PHP involves several steps. Below is a simplified example to help you understand the concept. Keep in mind that this example is not production-ready and lacks security measures that a robust system would need. It serves as a basic guide to get you started.
1. Folder Structure:
Create the following folder structure:
- images/
- level1/
- level2/
- level3/
- index.php
- authenticate.php
- success.php
- failure.php
2. Image Selection:
Place images in each level folder (level1/
, level2/
, level3/
). These images will be used for authentication.
3. index.php:
<?php
session_start();
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Image Authentication</title>
</head>
<body>
<h2>Image Authentication</h2>
<form action="authenticate.php" method="post">
<label for="level1">Select an image from Level 1:</label>
<select name="level1" id="level1">
<?php echo getImagesOptions('images/level1/'); ?>
</select>
<br>
<label for="level2">Select an image from Level 2:</label>
<select name="level2" id="level2">
<?php echo getImagesOptions('images/level2/'); ?>
</select>
<br>
<label for="level3">Select an image from Level 3:</label>
<select name="level3" id="level3">
<?php echo getImagesOptions('images/level3/'); ?>
</select>
<br>
<input type="submit" value="Authenticate">
</form>
<?php
function getImagesOptions($folder)
{
$options = "";
$files = glob($folder . '*.{jpg,jpeg,png,gif}', GLOB_BRACE);
foreach ($files as $file) {
$options .= "<option value='" . basename($file) . "'>" . basename($file) . "</option>";
}
return $options;
}
?>
</body>
</html>
4. authenticate.php:
<?php
session_start();
// Perform authentication logic
$level1Selection = $_POST['level1'];
$level2Selection = $_POST['level2'];
$level3Selection = $_POST['level3'];
// Implement your authentication logic here
// Compare selections against stored values or criteria
// Example: Check if all three selections are the same for simplicity
if ($level1Selection == $level2Selection && $level2Selection == $level3Selection) {
header("Location: success.php");
} else {
header("Location: failure.php");
}
?>
5. success.php and failure.php:
Create these pages to display success or failure messages accordingly.
This is a basic example, and for a production environment, you would need to implement additional security measures, such as hashing and salting passwords, protecting against SQL injection, and securing file uploads.