How to Build an AI-Powered Resume Builder Application using Nextjs, CopilotKit & OpenAI.
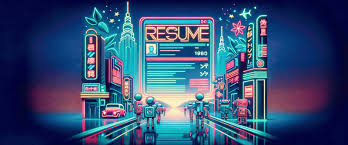
In this article, you will learn how to build an AI-powered resume builder application using Nextjs, CopilotKit & OpenAI.
We will cover:
- Leverage ChatGPT to write your resume & cover letter
- Gradually improve your resume by chatting with it
- Export your resume & cover letter into a PDF
CopilotKit: Build deeply integrated in-app AI chatbots
Just a quick background about us. CopilotKit is the open-source AI copilot platform. We make it easy to integrate powerful AI chatbots into your react apps. Fully customizable and deeply integrated.
Prerequisites
To get started with this tutorial, you need the following installed on your computer:
- A text editor (e.g., Visual Studio Code or any IDE)
- Node.js
- A package manager
Creating The Resume App Frontend With NextJS
Step 1: Open the command prompt and execute the following command.
Step 2: You will be prompted to select some options, as shown below.
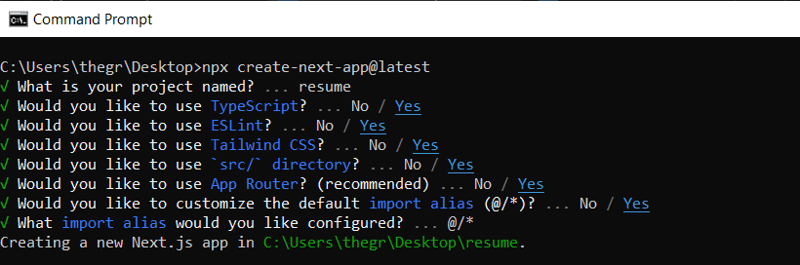
Step 3: Open the newly created Nextjs project using a text editor of your choice. Then, run the command below on the command line to install Preline UI with NextJS using Tailwind CSS. Follow this guide to complete the Preline setup.
npm install preline
Step 4: On the resume/app/page.tsx file, add the following code content
export default function Home() {
return (
<>
<header className="flex flex-wrap sm:justify-start sm:flex-nowrap z-50 w-full bg-slate-900 bg-gradient-to-b from-violet-600/[.15] via-transparent text-sm py-3 sm:py-0 dark:bg-gray-800 dark:border-gray-700">
<nav
className="relative max-w-7xl w-full mx-auto px-4 sm:flex sm:items-center sm:justify-between sm:px-6 lg:px-8 "
aria-label="Global">
<div className="flex items-center justify-between">
<a
className="flex-none text-xl text-gray-200 font-semibold dark:text-white py-8"
href="#"
aria-label="Brand">
ResumeBuilder
</a>
</div>
</nav>
</header>
{/* <!-- Hero --> */}
<div className="bg-slate-900 h-screen">
<div className="bg-gradient-to-b from-violet-600/[.15] via-transparent">
<div className="max-w-[85rem] mx-auto px-4 sm:px-6 lg:px-8 py-24 space-y-8">
{/* <!-- Title --> */}
<div className="max-w-3xl text-center mx-auto pt-10">
<h1 className="block font-medium text-gray-200 text-4xl sm:text-5xl md:text-6xl lg:text-7xl">
Craft A Compelling Resume With AI Resume Builder
</h1>
</div>
{/* <!-- End Title --> */}
<div className="max-w-3xl text-center mx-auto">
<p className="text-lg text-gray-400">
ResumeBuilder helps you create a resume that effectively
highlights your skills and experience.
</p>
</div>
{/* <!-- Buttons --> */}
<div className="text-center">
<a
className="inline-flex justify-center items-center gap-x-3 text-center bg-gradient-to-tl from-blue-600 to-violet-600 shadow-lg shadow-transparent hover:shadow-blue-700/50 border border-transparent text-white text-sm font-medium rounded-full focus:outline-none focus:ring-2 focus:ring-blue-600 focus:ring-offset-2 focus:ring-offset-white py-3 px-6 dark:focus:ring-offset-gray-800"
href="#">
Get started
<svg
className="flex-shrink-0 w-4 h-4"
xmlns="http://www.w3.org/2000/svg"
width="24"
height="24"
viewBox="0 0 24 24"
fill="none"
stroke="currentColor"
stroke-width="2"
stroke-linecap="round"
stroke-linejoin="round">
<path d="m9 18 6-6-6-6" />
</svg>
</a>
</div>
{/* <!-- End Buttons --> */}
</div>
</div>
</div>
{/* <!-- End Hero --> */}
</>
);
}
import { NextResponse } from "next/server";
import axios from "axios";
// Environment variables for GitHub API token and user details
const GITHUB_TOKEN = "Your GitHub personal access token";
const GITHUB_USERNAME = "Your GitHub account username";
// Axios instance for GitHub GraphQL API
const githubApi = axios.create({
baseURL: "https://api.github.com/graphql",
headers: {
Authorization: `bearer ${GITHUB_TOKEN}`,
"Content-Type": "application/json",
},
});
// GraphQL query to fetch user and repository data
const getUserAndReposQuery = `
query {
user(login: "${GITHUB_USERNAME}") {
name
email
company
bio
repositories(first: 3, orderBy: {field: CREATED_AT, direction: DESC}) {
edges {
node {
name
url
description
createdAt
... on Repository {
primaryLanguage{
name
}
stargazers {
totalCount
}
}
}
}
}
}
}
`;
// API route to handle resume data fetching
export async function GET(request: any) {
try {
// Fetch data from GitHub
const response = await githubApi.post("", { query: getUserAndReposQuery });
const userData = response.data.data.user;
// Format resume data
const resumeData = {
name: userData.name,
email: userData.email,
company: userData.company,
bio: userData.bio,
repositories: userData.repositories.edges.map((repo: any) => ({
name: repo.node.name,
url: repo.node.url,
created: repo.node.createdAt,
description: repo.node.description,
language: repo.node.primaryLanguage.name,
stars: repo.node.stargazers.totalCount,
})),
};
// Return formatted resume data
return NextResponse.json(resumeData);
} catch (error) {
console.error("Error fetching data from GitHub:", error);
return NextResponse.json({ message: "Internal Server Error" });
}
}