Software Projects
Text to Speech Converter Project Source Code
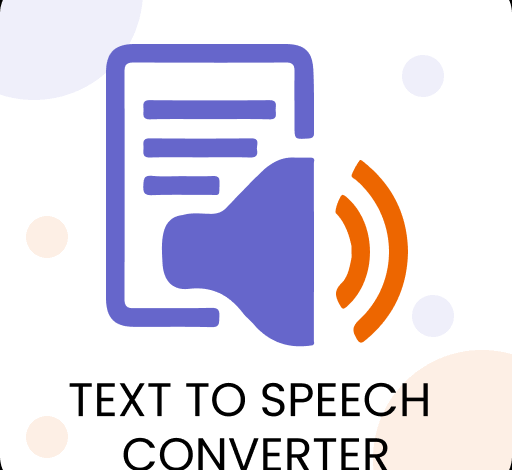
Text to speech converter Project source code: Creating a project that converts any text into speech could be an interesting and skill-pushing project while learning HTML CSS & JavaScriptToday in this blog you will learn to build a Text-to-Speech
Converter using HTML CSS & JavaScript. This converter will convert any text that will be typed in the input field. Recently I have provided a blog on how can we build a Calculator in HTML CSS & JavaScript, I believe that project will be also beneficial for you.
Steps for Creating a Text-to-Speech Converter in JavaScript
To create a Text-to-Speech Converter using HTML, CSS, and vanilla JavaScript, follow the given steps line by line:
- Create a folder. You can name this folder whatever you want, and inside this folder, create the mentioned files.
- Create an
index.html
file. The file name must be index and its extension .html - Create a
style.css
file. The file name must be style and its extension .css - Create a
script.js
file. The file name must be script and its extension .js
Index.html File
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Text to Speech Converter</title>
<link rel="stylesheet" href="style.css" />
</head>
<body>
<div class="container">
<header>Text to Speech Converter</header>
<textarea placeholder="Enter text"></textarea>
<button>Convert to Speech</button>
</div>
<script src="script.js" defer></script>
</body>
</html>
Styles.css
/* Import Google font - Poppins */
@import url("https://fonts.googleapis.com/css2?family=Poppins:wght@200;300;400;500;600;700&display=swap");
* {
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: "Poppins", sans-serif;
}
body {
height: 100vh;
display: flex;
align-items: center;
justify-content: center;
background: #87a5f8;
}
.container {
position: relative;
max-width: 350px;
width: 100%;
background: #fff;
border-radius: 12px;
padding: 20px;
box-shadow: 0 5px 10px rgba(0, 0, 0, 0.1);
}
header {
font-size: 18px;
color: #333;
font-weight: 500;
text-align: center;
}
textarea {
width: 100%;
height: 180px;
border-radius: 8px;
margin: 20px 0;
padding: 10px 15px;
resize: none;
outline: none;
border: 1px solid #aaa;
}
button {
width: 100%;
padding: 14px 0;
border: none;
border-radius: 8px;
color: #fff;
background: #6e93f7;
cursor: pointer;
transition: all 0.3s ease;
}
button:hover {
background: #4070f4;
}
Script.js File
const textarea = document.querySelector("textarea");
const button = document.querySelector("button");
let isSpeaking = true;
const textToSpeech = () => {
const synth = window.speechSynthesis;
const text = textarea.value;
if (!synth.speaking && text) {
const utternace = new SpeechSynthesisUtterance(text);
synth.speak(utternace);
}
if (text.length > 50) {
if (synth.speaking && isSpeaking) {
button.innerText = "Pause";
synth.resume();
isSpeaking = false;
} else {
button.innerText = "Resume";
synth.pause();
isSpeaking = true;
}
} else {
isSpeaking = false;
button.innerText = "Speaking";
}
setInterval(() => {
if (!synth.speaking && !isSpeaking) {
isSpeaking = true;
button.innerText = "Convert to Speech";
}
});
};
button.addEventListener("click", textToSpeech);