Download Any File From URL (Project Source Code)
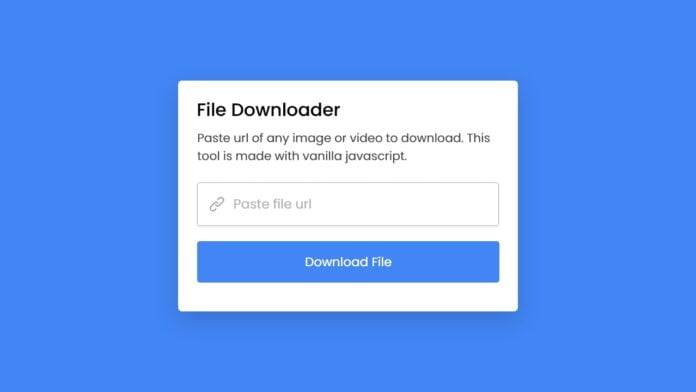
This tool (File Downloader) can download any file like image, video, pdf, svg, etc. Users have to paste a valid URL of the file and click the download button to download the file. Remember, the file should be publicly accessible to download.
This file downloader is made with pure JavaScript no server-side language is used to create it.
At first, I got the user entered file URL, and using fetch() API, I fetched the file. Once I got a response, I return the response as a blob() and in another then method, I got an object that contains details of the file.
Then, using URL.createObjectURL() method, I created a URL of that file object. This URL is stored in the document window. At last, I created a <a> tag and stored the URL as the href value of this tag, and click it so the file download. Watch the above video for a detailed explanation of each JavaScript line.
Note: If you get a cors (cross-origin resource sharing) error in the console during file downloading, that means the browser blocked the request because the requested site doesn’t allow you to access that
Index.html
<!DOCTYPE html>
<html lang="en" dir="ltr">
<head>
<meta charset="utf-8">
<title>File Downloader in JavaScript| CodingNepal</title>
<link rel="stylesheet" href="style.css">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
</head>
<body>
<div class="wrapper">
<header>
<h1>File Downloader</h1>
<p>Paste url of image, video, or pdf to download. This tool is made with vanilla javascript.</p>
</header>
<form action="#">
<input type="url" placeholder="Paste file url" required>
<button>Download File</button>
</form>
</div>
<script src="script.js"></script>
</body>
</html>
Styles.css
/* Import Google Font - Poppins */
@import url('https://fonts.googleapis.com/css2?family=Poppins:wght@400;500;600;700&display=swap');
*{
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: 'Poppins', sans-serif;
}
body{
display: flex;
padding: 0 10px;
align-items: center;
justify-content: center;
min-height: 100vh;
background: #4285F4;
}
.wrapper{
max-width: 500px;
background: #fff;
border-radius: 7px;
padding: 20px 25px 15px;
box-shadow: 0 15px 40px rgba(0,0,0,0.12);
}
header h1{
font-size: 27px;
font-weight: 500;
}
header p{
margin-top: 5px;
font-size: 18px;
color: #474747;
}
form{
margin: 20px 0 27px;
}
form input{
width: 100%;
height: 60px;
outline: none;
padding: 0 17px;
font-size: 19px;
border-radius: 5px;
border: 1px solid #b3b2b2;
transition: 0.1s ease;
}
form input::placeholder{
color: #b3b2b2;
}
form input:focus{
box-shadow: 0 3px 6px rgba(0,0,0,0.13);
}
form button{
width: 100%;
border: none;
opacity: 0.7;
outline: none;
color: #fff;
cursor: pointer;
font-size: 17px;
margin-top: 20px;
padding: 15px 0;
border-radius: 5px;
pointer-events: none;
background: #4285F4;
transition: opacity 0.15s ease;
}
form input:valid ~ button{
opacity: 1;
pointer-events: auto;
}
.js file
const fileInput = document.querySelector("input"),
downloadBtn = document.querySelector("button");
downloadBtn.addEventListener("click", e => {
e.preventDefault();
downloadBtn.innerText = "Downloading file...";
fetchFile(fileInput.value);
});
function fetchFile(url) {
fetch(url).then(res => res.blob()).then(file => {
let tempUrl = URL.createObjectURL(file);
const aTag = document.createElement("a");
aTag.href = tempUrl;
aTag.download = url.replace(/^.*[\\\/]/, '');
document.body.appendChild(aTag);
aTag.click();
downloadBtn.innerText = "Download File";
URL.revokeObjectURL(tempUrl);
aTag.remove();
}).catch(() => {
alert("Failed to download file!");
downloadBtn.innerText = "Download File";
});
}