JavaScript ProjectsSoftware Projects
Develop Analog Clock software (Source Code)
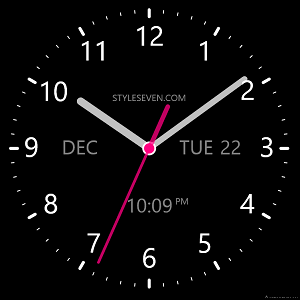
Creating an analog clock using HTML, CSS, and JavaScript is an better way to improve your coding skills and gain huge experience in web development.
The analog clock that we will develop will have a user interface design that is identical to the image provided. Furthermore, all of the HTML, CSS, and JavaScript code that I will utilize to construct this clock will be made available to you for free.
Steps to Develop an Analog Clock software
To create Analog Clock, follow the steps line by line:
- Create a folder. You can name this folder whatever you want, and inside this folder, create the mentioned files.
- Create an
index.html
file. The file name must be index and its extension .html - Create a
style.css
file. The file name must be style and its extension .css - Create a
script.js
file. The file name must be script and its extension.js
First, copy and paste the following codes into your index.html file.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Analog Clock JavaScript</title>
<link rel="stylesheet" href="style.css" />
<script src="script.js" defer></script>
</head>
<body>
<div class="container">
<div class="clock">
<label style="--i: 1"><span>1</span></label>
<label style="--i: 2"><span>2</span></label>
<label style="--i: 3"><span>3</span></label>
<label style="--i: 4"><span>4</span></label>
<label style="--i: 5"><span>5</span></label>
<label style="--i: 6"><span>6</span></label>
<label style="--i: 7"><span>7</span></label>
<label style="--i: 8"><span>8</span></label>
<label style="--i: 9"><span>9</span></label>
<label style="--i: 10"><span>10</span></label>
<label style="--i: 11"><span>11</span></label>
<label style="--i: 12"><span>12</span></label>
<div class="indicator">
<span class="hand hour"></span>
<span class="hand minute"></span>
<span class="hand second"></span>
</div>
</div>
<div class="mode-switch">Dark Mode</div>
</div>
</body>
</html>
/* Import Google font - Poppins */
@import url("https://fonts.googleapis.com/css2?family=Poppins:wght@200;300;400;500;600;700&display=swap");
* {
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: "Poppins", sans-serif;
}
:root {
--primary-color: #f6f7fb;
--white-color: #fff;
--black-color: #18191a;
--red-color: #e74c3c;
}
body {
display: flex;
min-height: 100vh;
align-items: center;
justify-content: center;
background: var(--primary-color);
}
body.dark {
--primary-color: #242526;
--white-color: #18191a;
--black-color: #fff;
--red-color: #e74c3c;
}
.container {
display: flex;
flex-direction: column;
align-items: center;
gap: 60px;
}
.container .clock {
display: flex;
height: 400px;
width: 400px;
border-radius: 50%;
align-items: center;
justify-content: center;
background: var(--white-color);
box-shadow: 0 15px 25px rgba(0, 0, 0, 0.1), 0 25px 45px rgba(0, 0, 0, 0.1);
position: relative;
}
.clock label {
position: absolute;
inset: 20px;
text-align: center;
transform: rotate(calc(var(--i) * (360deg / 12)));
}
.clock label span {
display: inline-block;
font-size: 30px;
font-weight: 600;
color: var(--black-color);
transform: rotate(calc(var(--i) * (-360deg / 12)));
}
.container .indicator {
position: absolute;
height: 10px;
width: 10px;
display: flex;
justify-content: center;
}
.indicator::before {
content: "";
position: absolute;
height: 100%;
width: 100%;
border-radius: 50%;
z-index: 100;
background: var(--black-color);
border: 4px solid var(--red-color);
}
.indicator .hand {
position: absolute;
height: 130px;
width: 4px;
bottom: 0;
border-radius: 25px;
transform-origin: bottom;
background: var(--red-color);
}
.hand.minute {
height: 120px;
width: 5px;
background: var(--black-color);
}
.hand.hour {
height: 100px;
width: 8px;
background: var(--black-color);
}
.mode-switch {
padding: 10px 20px;
border-radius: 8px;
font-size: 22px;
font-weight: 400;
display: inline-block;
color: var(--white-color);
background: var(--black-color);
box-shadow: 0 5px 10px rgba(0, 0, 0, 0.1);
cursor: pointer;
}
// Get references to DOM elements
const body = document.querySelector("body"),
hourHand = document.querySelector(".hour"),
minuteHand = document.querySelector(".minute"),
secondHand = document.querySelector(".second"),
modeSwitch = document.querySelector(".mode-switch");
// check if the mode is already set to "Dark Mode" in localStorage
if (localStorage.getItem("mode") === "Dark Mode") {
// add "dark" class to body and set modeSwitch text to "Light Mode"
body.classList.add("dark");
modeSwitch.textContent = "Light Mode";
}
// add a click event listener to modeSwitch
modeSwitch.addEventListener("click", () => {
// toggle the "dark" class on the body element
body.classList.toggle("dark");
// check if the "dark" class is currently present on the body element
const isDarkMode = body.classList.contains("dark");
// set modeSwitch text based on "dark" class presence
modeSwitch.textContent = isDarkMode ? "Light Mode" : "Dark Mode";
// set localStorage "mode" item based on "dark" class presence
localStorage.setItem("mode", isDarkMode ? "Dark Mode" : "Light Mode");
});
const updateTime = () => {
// Get current time and calculate degrees for clock hands
let date = new Date(),
secToDeg = (date.getSeconds() / 60) * 360,
minToDeg = (date.getMinutes() / 60) * 360,
hrToDeg = (date.getHours() / 12) * 360;
// Rotate the clock hands to the appropriate degree based on the current time
secondHand.style.transform = `rotate(${secToDeg}deg)`;
minuteHand.style.transform = `rotate(${minToDeg}deg)`;
hourHand.style.transform = `rotate(${hrToDeg}deg)`;
};
// call updateTime to set clock hands every second
setInterval(updateTime, 1000);
//call updateTime function on page load
updateTime();