User Registration & Login and User Management System With Admin Panel
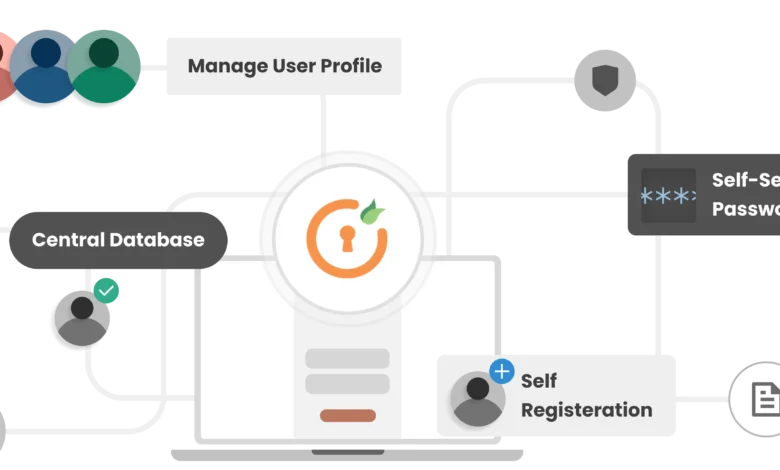
In this sure digital age, the need for robust and big user registration, login, and management systems is highest for any web application.
A user registration and login system is the gateway to a personalized user experience. It allows users to create accounts, access exclusive content, and manage their profiles.
Key Components
- User Registration
- User Login
- User Management System
- Admin Panel
1. User Registration
Frontend Form
The registration process begins with a frontend form where users input their details. Common fields include username, email, password, and confirmation password. Here is a basic example in HTML:
<form method="POST" action="/register">
<input type="text" name="username" placeholder="Username" required>
<input type="email" name="email" placeholder="Email" required>
<input type="password" name="password" placeholder="Password" required>
<input type="password" name="confirm_password" placeholder="Confirm Password" required>
<button type="submit">Register</button>
</form>
Backend Processing
Upon form submission, the backend processes the data by validating inputs, hashing passwords, and storing user information in the database. Using Django as an example:
# models.py
from django.contrib.auth.models import AbstractUser
class CustomUser(AbstractUser):
pass
# forms.py
from django import forms
from django.contrib.auth.forms import UserCreationForm
from .models import CustomUser
class CustomUserCreationForm(UserCreationForm):
class Meta:
model = CustomUser
fields = ('username', 'email')
# views.py
from django.shortcuts import render, redirect
from .forms import CustomUserCreationForm
def register(request):
if request.method == 'POST':
form = CustomUserCreationForm(request.POST)
if form.is_valid():
form.save()
return redirect('login')
else:
form = CustomUserCreationForm()
return render(request, 'register.html', {'form': form})
2. User Login
Frontend Form
Similar to registration, the login form requires the user’s credentials.
<form method="POST" action="/login">
<input type="text" name="username" placeholder="Username" required>
<input type="password" name="password" placeholder="Password" required>
<button type="submit">Login</button>
</form>
Backend Processing
The backend authenticates the user credentials and initiates a session.
# views.py
from django.contrib.auth import authenticate, login
from django.shortcuts import render, redirect
def login_view(request):
if request.method == 'POST':
username = request.POST['username']
password = request.POST['password']
user = authenticate(request, username=username, password=password)
if user is not None:
login(request, user)
return redirect('home')
else:
return render(request, 'login.html', {'error': 'Invalid credentials'})
return render(request, 'login.html')
3. User Management System
Profile Management
Users should be able to update their profile information and change their passwords.
# views.py
from django.contrib.auth.decorators import login_required
from django.contrib.auth.forms import PasswordChangeForm
from django.contrib.auth import update_session_auth_hash
@login_required
def profile_view(request):
if request.method == 'POST':
form = PasswordChangeForm(request.user, request.POST)
if form.is_valid():
user = form.save()
update_session_auth_hash(request, user)
return redirect('profile')
else:
form = PasswordChangeForm(request.user)
return render(request, 'profile.html', {'form': form})
4. Admin Panel
Admin Interface
An admin panel allows administrators to manage user accounts, monitor activities, and enforce policies. Using Django’s built-in admin interface simplifies this process.
# admin.py
from django.contrib import admin
from .models import CustomUser
admin.site.register(CustomUser)
Custom Dashboard
For more control, a custom admin dashboard can be built with features like user analytics, role management, and system.