JavaScript ProjectsSoftware Projects
How To Do Auto Search Using JavaScript
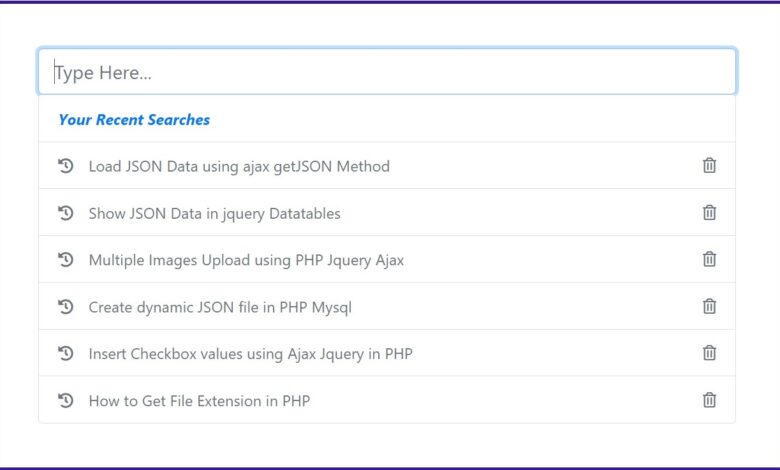
In this code we will tackle about Auto Search using JavaScript. The program will enable to automatically search a row data. You are free to modify, and use this code. To learn more about this, just follow the steps below.
Getting started:
First you have to download bootstrap framework, this is the link for the bootstrap that I used for the layout design https://getbootstrap.com/.
The Main Interface
This code contains the interface of the application. To create this just write these block of code inside the text editor and save this as index.html.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
<link rel="stylesheet" type="text/css" href="css/bootstrap.css"/>
</head>
<body>
<nav class="navbar navbar-default">
<div class="container-fluid">
<a class="navbar-brand" href="https://sourcecodester.com">Sourcecodester</a>
</div>
</nav>
<div class="col-md-3"></div>
<div class="col-md-6 well">
<h3 class="text-primary">Auto Search Using JavaScript</h3>
<hr style="border-top:1px dotted #ccc;"/>
<div class="col-md-4">
<div class="form-group">
<label>Enter a name</label>
<div class="input-group">
<div class="input-group-addon"><span class="glyphicon glyphicon-search"></span></div>
<input type="text" id="search" placeholder="Search here..." onkeyup="autoSearch()" class="form-control"/>
</div>
</div>
</div>
<table class="table table-bordered">
<thead class="alert-info">
<tr>
<th>Member List</th>
</tr>
</thead>
<tbody id="result"></tbody>
</table>
<ul class="list-group" id="list"></ul>
</div>
<script src="js/script.js"></script>
</body>
</html>
Creating the Script
This code contains the script of the application. The code will automatically search a specific keyword. To do this just copy and write these block of codes inside the text editor, then save it as script.js inside the js folder.
var members = ['Tony Stark', 'Jin Mori', 'Luke Cage'];
displayMember();
function displayMember(){
members.sort(function(a,b){
if(a < b){
return -1;
}
if(a > b){
return 1;
}
return 0;
});
data = "";
for(var i=0; i < members.length; i++){
data += "<tr><td>"+members[i]+"</td></tr>";
}
document.getElementById('result').innerHTML = data;
}
function autoSearch(){
var search=document.getElementById('search').value.toLowerCase();
var parent=document.getElementById("result");
var children=parent.getElementsByTagName('tr');
for(var i=0; i<children.length; i++){
var keyword=children[i].innerText;
if(keyword.toLowerCase().indexOf(search)>-1){
children[i].style.display="";
}else{
children[i].style.display = "none";
}
}
}