Laundry Management System in PHP MySQL Source Code
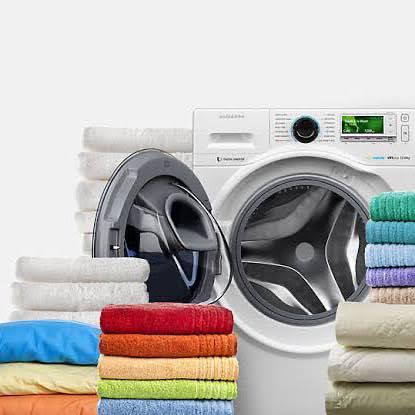
develop in PHP MySQL database, using HTML, CSS, JavaScript, Modal, and bootstrap. This system contains of admin and staff, the admin can manage the staff like delete, update, add new user, and update information, the staff can manage selected data control by the admin, and also the system have an own inventory, this system is friendly user and easy to understand.
Online Laundry Management System is a robust application developed using PHP and MySQL database. It incorporates essential web technologies such as HTML, CSS, JavaScript, Modal, and Bootstrap to ensure a seamless user experience. This system caters to both administrators and staff members, offering a wide range of functionalities to streamline laundry operations.
Features of the Project
- Dashboard
- Home
- Laundry List
- Laundry Category
- Supply List
- Reports
- Users
- Total Profit Today,
- Total Customer Today
- Total Claimed Laundry Today
Creating a Laundry Management System in PHP and MySQL involves designing and implementing a system to manage laundry orders, customers, inventory, staff, and billing. Below is a step-by-step guide to help you build this system:
1. Database Design:
- Design your MySQL database schema to store information about orders, customers, inventory, staff, and billing.
- Create tables for
orders
,customers
,inventory
,staff
,billing
, and establish relationships between them (e.g., an order is associated with a customer and staff member, inventory items are associated with orders, etc.).
2. Setting Up the Development Environment:
- Set up a local development environment with PHP and MySQL, using tools like XAMPP or MAMP.
- Create a new database and import your database schema using SQL scripts.
3. User Authentication:
- Implement user authentication functionality to allow staff members to log in securely.
- Use PHP sessions or JSON Web Tokens (JWT) for user authentication and authorization.
4. Order Management:
- Develop functionality for creating, editing, and managing laundry orders, including order details, customer information, order status, etc.
- Implement features for assigning orders to staff members and tracking order progress.
5. Customer Management:
- Implement features for managing customer records, including adding, editing, and deleting customer profiles.
- Track customer preferences, contact information, order history, and payment details.
6. Inventory Management:
- Develop functionality for managing laundry inventory, including tracking stock levels, adding new items, and updating item quantities.
- Implement features for automatically deducting inventory items when orders are processed.
7. Staff Management:
- Implement features for managing staff records, including adding, editing, and deleting staff profiles.
- Assign staff roles and responsibilities, track work schedules, and monitor staff performance.
8. Billing and Invoicing:
- Develop functionality for generating invoices and billing statements for laundry services.
- Calculate fees based on order details such as item quantity, service type, delivery options, etc.
9. Reporting and Analytics:
- Develop reporting tools to generate summary reports and analytics on order volume, revenue, inventory turnover, staff productivity, etc.
- Generate dynamic reports using PHP and MySQL queries, and display them in tabular or graphical formats.
10. Testing and Debugging:
- Thoroughly test each feature of your laundry management system to ensure it works as expected. Pay attention to edge cases and error handling scenarios.
- Use tools like PHPUnit for automated testing and debugging tools like Xdebug for troubleshooting.
11. Deployment:
- Deploy your laundry management system to a live web server, ensuring it meets the necessary requirements for PHP and MySQL.
- Secure your application by implementing measures such as HTTPS encryption, input validation, and SQL injection prevention.
By following these steps and considering these tips, you can develop a comprehensive Laundry Management System in PHP and MySQL that meets the needs of laundry businesses and service providers.
Creating a laundry management system in PHP involves designing and implementing various functionalities such as managing orders, customers, inventory, staff, and billing. Below is an overview of how you can structure the PHP code for a laundry system:
1. Database Connection:
<?php
$servername = "localhost";
$username = "username";
$password = "password";
$dbname = "laundry_system";
// Create connection
$conn = new mysqli($servername, $username, $password, $dbname);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
?>
2. Managing Orders:
// Add new order
function addOrder($customer_id, $items, $pickup_date, $delivery_date) {
// Insert order details into the database
}
// Update order status
function updateOrderStatus($order_id, $status) {
// Update order status in the database
}
// Get orders by status
function getOrdersByStatus($status) {
// Retrieve orders from the database based on status
}
3. Managing Customers:
// Add new customer
function addCustomer($name, $phone, $email) {
// Insert customer details into the database
}
// Update customer details
function updateCustomer($customer_id, $name, $phone, $email) {
// Update customer details in the database
}
// Get customer by ID
function getCustomerByID($customer_id) {
// Retrieve customer details from the database
}
4. Managing Inventory:
// Add new item to inventory
function addItemToInventory($name, $quantity, $price) {
// Insert item details into the database
}
// Update item quantity
function updateItemQuantity($item_id, $quantity) {
// Update item quantity in the database
}
// Get item by ID
function getItemByID($item_id) {
// Retrieve item details from the database
}
5. Managing Staff:
// Add new staff member
function addStaffMember($name, $role, $phone, $email) {
// Insert staff member details into the database
}
// Update staff member details
function updateStaffMember($staff_id, $name, $role, $phone, $email) {
// Update staff member details in the database
}
// Get staff member by ID
function getStaffMemberByID($staff_id) {
// Retrieve staff member details from the database
}
6. Billing and Invoicing:
// Generate invoice for order
function generateInvoice($order_id) {
// Calculate total amount based on order details
// Generate invoice PDF or HTML
}
7. Reporting and Analytics:
// Generate report on order volume, revenue, etc.
function generateReport() {
// Retrieve data from the database and format into a report
}
This is just a basic structure, and you’ll need to fill in the actual implementation details for each function based on your specific requirements and database schema. Additionally, consider implementing security measures such as input validation, sanitization, and prepared statements to prevent SQL injection and other security vulnerabilities.
How to Run
Above all, to run this project you must have installed a virtual server i.e XAMPP on your PC. Online Laundry Management System in PHP and MySQL with source code is free to download, Use for educational purposes only!
Follow the following steps after Starting Apache and MySQL in XAMPP:
1st Step: Firstly, Extract the file
2nd Step: After that, Copy the main project folder
3rd Step: So, you need to Paste in xampp/htdocs/
Further, Now Connecting Database
4th Step: So, for now, Open a browser and go to URL “http://localhost/phpmyadmin/”
5th Step: After that, Click on the databases tab
6th Step: So, Create a database naming “laundry_db” and then click on the import tab
7th Step: Certainly, Click on browse file and select “laundry_db.sql” file which is inside the “db” folder
8th Step: Meanwhile, click on Go button.
After Creating Database,
9th Step: Moreover, Open a browser and go to URL “http://localhost/Laundry_Management_System”