JavaScript ProjectsSoftware Projects
Best JavaScript Project Ideas with source code
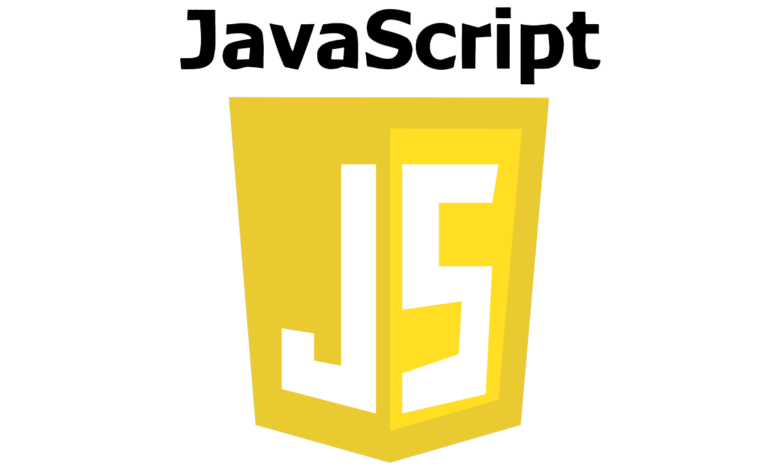
Here are top JavaScript project ideas that can help you improve your coding knowledge and add impressive projects to your portfolio:
1. To-Do List Application
- Filter tasks by status (all, active, completed).
- Store tasks in local storage to persist data across sessions.
2. Weather App
- Fetch weather data from an API (e.g., OpenWeatherMap).
- Display current weather conditions based on the user’s location or search query.
- Show a 5-day weather forecast.
3. Interactive Quiz Game
- Multiple-choice questions with a timer.
- Display score and correct answers at the end.
- Store high scores using local storage.
4. REAL TIME Chat Application
- Real-time messaging using WebSockets (e.g., Socket.IO).
- User authentication and chat rooms.
- Display online/offline status of users.
5. Expense Tracker
- Track income and expenses with categories.
- Display a summary of total income, expenses, and balance.
- Visualize data with charts and graphs.
6. Recipe Finder
- Search for recipes based on ingredients or dish name.
- Fetch and display recipes from an API (e.g., TheMealDB or Edamam).
- Show detailed recipe instructions, ingredients, and nutritional information.
7. E-commerce Product Page
- Display products with images, descriptions, and prices.
- Implement a shopping cart with add/remove items functionality.
- Calculate total price and handle checkout process.
8. Movie Database App
- Search for movies and display detailed information using an API (e.g., OMDb API or TMDb API).
- Show movie ratings, reviews, cast, and trailers.
- Allow users to save their favorite movies.
9. Drawing App
- Implement a canvas where users can draw with different colors and brush sizes.
- Allow users to save their drawings as images.
- Add features like undo/redo and clear canvas.
10. Portfolio Website
- Showcase your projects, skills, and experience.
- Include sections like About, Projects, Blog, and Contact.
Coding Example Implementation: To-Do List Application
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>To-Do List</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="container">
<h1>To-Do List</h1>
<form id="task-form">
<input type="text" id="task-input" placeholder="Add a new task" required>
<button type="submit">Add Task</button>
</form>
<ul id="task-list"></ul>
</div>
<script src="script.js"></script>
</body>
</html>
CSS (styles.css):
body {
font-family: Arial, sans-serif;
background: #f4f4f4;
margin: 0;
padding: 0;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
.container {
background: #fff;
padding: 20px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
border-radius: 5px;
width: 300px;
}
h1 {
text-align: center;
}
form {
display: flex;
}
input[type="text"] {
flex: 1;
padding: 10px;
border: 1px solid #ccc;
border-radius: 5px 0 0 5px;
}
button {
padding: 10px;
border: 1px solid #ccc;
background: #28a745;
color: #fff;
cursor: pointer;
border-radius: 0 5px 5px 0;
}
ul {
list-style: none;
padding: 0;
margin-top: 20px;
}
li {
background: #f4f4f4;
padding: 10px;
margin-bottom: 10px;
display: flex;
justify-content: space-between;
border-radius: 5px;
}
li.completed {
text-decoration: line-through;
color: #aaa;
}
JavaScript (script.js):
document.addEventListener('DOMContentLoaded', () => {
const taskForm = document.getElementById('task-form');
const taskInput = document.getElementById('task-input');
const taskList = document.getElementById('task-list');
taskForm.addEventListener('submit', addTask);
taskList.addEventListener('click', handleTaskActions);
loadTasks();
function addTask(event) {
event.preventDefault();
const taskText = taskInput.value;
if (taskText) {
const taskItem = createTaskElement(taskText);
taskList.appendChild(taskItem);
saveTask(taskText);
taskInput.value = '';
}
}
function handleTaskActions(event) {
if (event.target.classList.contains('delete')) {
const taskItem = event.target.parentElement;
taskItem.remove();
deleteTask(taskItem.innerText);
} else if (event.target.classList.contains('toggle-complete')) {
const taskItem = event.target.parentElement;
taskItem.classList.toggle('completed');
updateTaskStatus(taskItem.innerText);
}
}
function createTaskElement(taskText) {
const li = document.createElement('li');
li.innerText = taskText;
const deleteButton = document.createElement('button');
deleteButton.innerText = 'Delete';
deleteButton.classList.add('delete');
li.appendChild(deleteButton);
const toggleCompleteButton = document.createElement('button');
toggleCompleteButton.innerText = 'Complete';
toggleCompleteButton.classList.add('toggle-complete');
li.appendChild(toggleCompleteButton);
return li;
}
function saveTask(taskText) {
let tasks = getTasksFromLocalStorage();
tasks.push({ text: taskText, completed: false });
localStorage.setItem('tasks', JSON.stringify(tasks));
}
function deleteTask(taskText) {
let tasks = getTasksFromLocalStorage();
tasks = tasks.filter(task => task.text !== taskText);
localStorage.setItem('tasks', JSON.stringify(tasks));
}
function updateTaskStatus(taskText) {
let tasks = getTasksFromLocalStorage();
tasks = tasks.map(task => {
if (task.text === taskText) {
task.completed = !task.completed;
}
return task;
});
localStorage.setItem('tasks', JSON.stringify(tasks));
}
function getTasksFromLocalStorage() {
const tasks = localStorage.getItem('tasks');
return tasks ? JSON.parse(tasks) : [];
}
function loadTasks() {
const tasks = getTasksFromLocalStorage();
tasks.forEach(task => {
const taskItem = createTaskElement(task.text);
if (task.completed) {
taskItem.classList.add('completed');
}
taskList.appendChild(taskItem);
});
}
});
Summary
These projects will help you gain practical experience with JavaScript, including DOM manipulation, API integration, state management, and more. Each project provides opportunities to learn and apply different aspects of JavaScript and web development. Start with simpler projects like the To-Do List Application and gradually move on to more complex ones like the Chat Application or Weather App.