Anime and Cartoon Photo Gallery from image directory in PHP
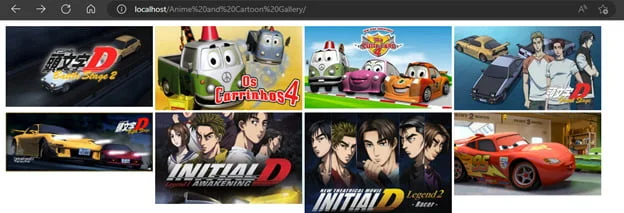
Anime and Cartoon Photo Gallery from image directory in PHP When you wish to make a photo gallery out of a sizable number of image files in a directory, this technique is advised.
1. Download and Include
The gallery was created using the simplelightbox jQuery library, which can be downloaded here.
Include simplelightbox.min.css and simple-lightbox.jquery.min.js.
<link href='simplelightbox-master/dist/simplelightbox.min.css' rel='stylesheet' type='text/css'>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script type="text/javascript" src="simplelightbox-master/dist/simple-lightbox.jquery.min.js"></script>
2. HTML and PHP
The files are sourced from the images directory which also has a sub-directory thumbnail.
Specified valid image extensions in the $image_extensions Array variable and target directory in $dir.
Read every file in the directory, then establish the paths for the thumbnails and images.
Use the path in the image source if the file is a genuine image and not a directory.
For this tutorial, we use $count variable to show 4 images in a row.
Finished Code
<div class='container'>
<div class="gallery">
<?php
// Image extensions
$image_extensions = array("png","jpg","jpeg","gif");
// Target directory
$dir = 'images/';
if (is_dir($dir)){
if ($dh = opendir($dir)){
$count = 1;
// Read files
while (($file = readdir($dh)) !== false){
if($file != '' && $file != '.' && $file != '..'){
// Thumbnail image path
$thumbnail_path = "images/thumbnail/".$file;
// Image path
$image_path = "images/".$file;
$thumbnail_ext = pathinfo($thumbnail_path, PATHINFO_EXTENSION);
$image_ext = pathinfo($image_path, PATHINFO_EXTENSION);
// Check its not folder and it is image file
if(!is_dir($image_path) &&
in_array($thumbnail_ext,$image_extensions) &&
in_array($image_ext,$image_extensions)){
?>
<!-- Image -->
<a href="<?php echo $image_path; ?>">
<img src="<?php echo $thumbnail_path; ?>" alt="" title=""/>
</a>
<!-- --- -->
<?php
// Break
if( $count%4 == 0){
?>
<div class="clear"></div>
<?php
}
$count++;
}
}
}
closedir($dh);
}
}
?>
</div>
</div>
CSS
.container .gallery a img {
float: left;
width: 20%;
height: auto;
border: 2px solid #fff;
-webkit-transition: -webkit-transform .15s ease;
-moz-transition: -moz-transform .15s ease;
-o-transition: -o-transform .15s ease;
-ms-transition: -ms-transform .15s ease;
transition: transform .15s ease;
position: relative;
}
.container .gallery a:hover img {
-webkit-transform: scale(1.05);
-moz-transform: scale(1.05);
-o-transform: scale(1.05);
-ms-transform: scale(1.05);
transform: scale(1.05);
z-index: 5;
}
.clear {
clear: both;
float: none;
width: 100%;
}
JQuery
<!-- Script -->
<script type='text/javascript'>
$(document).ready(function(){
// Intialize gallery
var gallery = $('.gallery a').simpleLightbox();
});
</script>